In this first part of our two-part series, we will build a basic HTTP server in Java using TCP network programming. We will start by setting up the server socket and then delve into the specifics of HTTP request handling, focusing on parsing and responding to a simple HTTP GET request.
Getting Started: Setting Up Your Development Environment
Before we begin coding, make sure you have the following:
- Java Development Kit (JDK): Install JDK 8 or later to compile and run Java applications.
- IDE or Text Editor: You can use any IDE like IntelliJ IDEA, Eclipse, or VSCode for Java development.
- Basic Understanding of Java: Familiarity with Java programming is essential as we will focus on networking concepts rather than basic syntax.
Understanding the Components of an HTTP Request
HTTP requests are fundamental to web communication, allowing clients to request resources from servers. Each HTTP request consists of several key components:
- Request Line
- Headers
- Blank Line A single blank line (carriage return and line feed) that separates headers from the body. This is crucial as it tells the server where the headers end and the body begins.
- and an Optional Body
Here’s a detailed breakdown of each part:
1. Request Line
The request line is the first line of any HTTP request. It contains three crucial pieces of information:
- Method: This indicates the action the client wants to perform. Common methods include
GET
(retrieve data),POST
(submit data),PUT
(update data), andDELETE
(remove data). - Path: The URL path to the resource on the server. This does not include the domain name but often includes the directory structure on the server and any query parameters.
- HTTP Version: Specifies the version of the HTTP protocol (e.g., HTTP/1.1, HTTP/2).
Example of a request line:
GET /index.html HTTP/1.1
In this example:
- The method is
GET
, indicating a request to retrieve data. - The path is
/index.html
, specifying what resource is requested. - The HTTP version is
1.1
, determining the protocol’s rules to be followed.
2. Headers
Headers provide additional information about the request. They can specify how the client wants to receive data, the type of data being sent, authentication information, and more. Headers are key-value pairs, and each header is on a new line. Some common request headers include:
- Host: The domain name of the server (required in HTTP/1.1).
- User-Agent: Information about the client software making the request.
- Accept: The types of content that the client can understand.
- Content-Type: Indicates the type of data in the body of the request (used with POST and PUT requests).
- Authorization: Credentials for authenticating the client to the server.
Example of headers in a request:
Host: www.example.com User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.36 Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8
3. Body
The body of the request is optional and not included in all requests. It is primarily used with methods like POST
and PUT
, where the client sends data to the server as part of the request. The body can contain data in various formats, such as form data, JSON, or XML, depending on what the server accepts and what the headers declare.
Example of a body in a POST request:
username=example&password=12345
This body is x-www-form-urlencoded
, which is common for submitting form data.
Http Request Example
POST /login HTTP/1.1 Host: www.example.com Content-Type: application/x-www-form-urlencoded Content-Length: 37 User-Agent: Mozilla/5.0 (compatible; MSIE 10.0; Windows NT) Accept: text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8 username=exampleUser&password=examplePass
HTTP Response Specifications
Responding to an HTTP request in a particular format is essential for ensuring that clients, such as web browsers or other HTTP clients, can understand and correctly process the response.
Basic Structure of an HTTP Response
An HTTP response consists of the following:
- Status Line: Includes the HTTP version, status code, and a status message.
- Headers: Provide additional information about the response, like content type and length.
- Blank Line: Separates headers from the body.
- Body: The actual content of the response.
Here’s why each part is essential:
- Status Line: Tells the client about the result of the request. For example, “200 OK” means the request was successfully processed.
- Headers:
Content-Type
tells the client what the type of the returned content is, which is necessary for the client to render it appropriately. Other headers can dictate caching policies, character set, and more. - Blank Line: A single blank line (carriage return and line feed) that separates headers from the body. This is crucial as it tells the client where the headers end and the body begins.
- Body: This is the content that the client asked for. It could be HTML, JSON, an image, or any other type of data.
Http Response Example
HTTP/1.1 200 OK Content-Type: text/html Content-Length: 44 <html><body><h1>Hello, World!</h1></body></html>
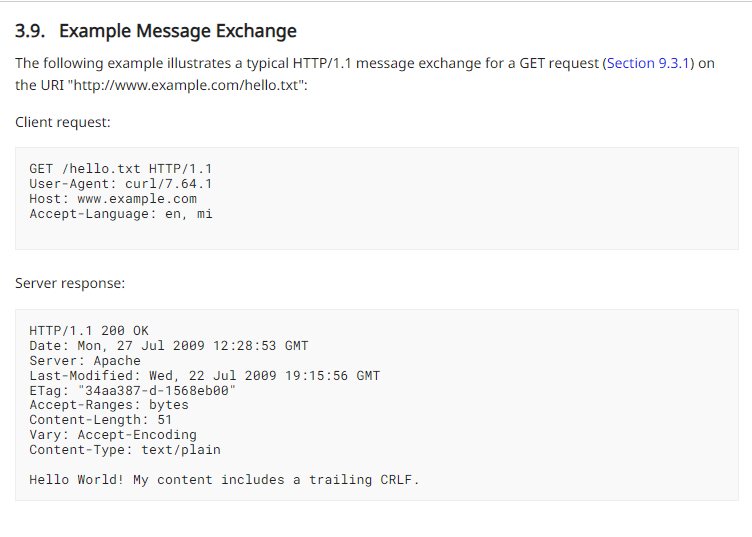
Conclusion
In the first part of our blog series, we explored the HTTP specification, detailing the structure and components of HTTP requests and responses. For those interested in delving deeper, you can refer to the HTTP RFC for comprehensive insights and official guidelines. An illustrative example of an HTTP request and response exchange is available in the RFC documentation, which can be accessed here. This resource is invaluable for understanding the practical implementation of the concepts discussed and for enhancing your knowledge of network communications within the HTTP framework.
Preview of Part 2
In the upcoming second part of this blog series, we will apply our knowledge of HTTP specifications to build a simple HTTP server using Java. We’ll focus exclusively on Java’s built-in network programming capabilities, without relying on any external libraries. Additionally, we’ll demonstrate how to interact with our server using a web browser to receive actual HTTP responses. This practical application will solidify your understanding of both Java network programming and HTTP communication fundamentals.
Check out part 2 of blog here: Building a Simple HTTP Server in Java with TCP Network Programming
[…] the first part of this blog series, Simple HTTP Server in Java with TCP Network Programming, we discussed the specifications of HTTP requests and responses according to the RFC standards, and […]